In this article, I am going to discuss the use and need of the keywords const and read-only in C#. Please read our previous article before proceeding to this article where we discussed the Static and Non-Static Members in C# with some examples. The const and read-only are very two useful keywords in C# and also little confusing to understand. So, as part of this article, we are going to discuss the following pointers in details.
- Const Variable in C#.
- Example using Const variable.
- Read-Only variable in C#.
- Example using the readonly variable.
- Difference between Const, Readonly, Static and non-static variable in C#.
According to MSDN
The Constants are the immutable values which are known at the time of program compilation and do not change their values for the lifetime of the program.
The Read-only variables are also immutable values but these values are known at runtime and also do not change their values for the life of the program.
With the above definition in mind, let’s try to understand the const and readonly with some example.
Const Variable in C#:
- The keyword const is used to create a “constant” variable. It means it will create a variable whose value is never going to be changed. In simple word, we can say that the variable whose value cannot be changed or modified once after its declaration is known as a constant variable.
- Constants are static by default.
- It is mandatory to initialize a constant variable at the times of its declaration.
- The behavior of a constant variable is same as the behavior of static variable i.e. maintains only one copy in the life cycle of class and initialize immediately once the execution of the class start (object not required)
- The only difference between a static and constant variable is that the static variable value can be modified but a constant variable value can never be modified.
Note: As we already discussed constants variable should be assigned a value at the time of variable declaration and hence these values are known at compile time. So, whenever we declare a constant variable, the C# compiler substitutes its value directly into the Intermediate Language (MSIL).
Example: Let us understand the Const keyword with one example.
- namespace ConstDemo
- {
- class ConstExample
- {
- //we need to assign a value to the const variable
- //at the time of const variable declaration else it will
- //give compile time error
- public const int number = 5;
- }
- class Program
- {
- static void Main(string[] args)
- {
- //Const variables are static in nature
- //so we can access them by using class name
- Console.WriteLine(ConstExample.number);
- //We can also declare constant variable within a function
- const int no = 10;
- Console.WriteLine(no);
- //Once after declaration we cannot change the value
- //of a constant variable. so the below live gives error
- //no = 20;
- Console.WriteLine("Press any key to exist.");
- Console.ReadLine();
- }
- }
- }
Read-only Variable in C#:
- The variable which is declared by using the readonly keyword is known as a read-only variable. The read-only variable’s value cannot be modified once after its initialization.
- It is not mandatory or required to initialize the read-only variable at the time of its declaration like a constant. You can initialize the read-only variables under a constructor but the most important point is that once after initialization, you cannot modify the value.
- The behavior of a read-only variable is similar to the behavior of a non-static variable. That is, it maintains a separate copy for each object. The only difference between these two is non-static variables can be modified while the read-only variables cannot be modified.
- A constant variable is a fixed value for the complete class whereas a read-only variable is a fixed value but specific to one object of the class.
Example: Let us understand Read-only in C# variable with one example.
- namespace ReadOnlyDemo
- {
- class ReadOnlyExample
- {
- public readonly int number = 5;
- }
- class Program
- {
- static void Main(string[] args)
- {
- ReadOnlyExample readOnlyInstance = new ReadOnlyExample();
- Console.WriteLine(readOnlyInstance.number);
- Console.WriteLine("Press any key to exist.");
- Console.ReadLine();
- }
- }
- }
In the above example, the read-only variable is assigned with a value at the time of its declaration and is accessed using the instance of the class rather than using the class name as read-only variables are non-static in nature.
Suppose, you may have another instance of the class, which might have the read-only number variable assigned to a different value based on some conditions. Can I do it? Yes, because the read-only variables are known at runtime.
Let understand this with one example.
- namespace ReadOnlyDemo
- {
- class ReadOnlyExample
- {
- //You can initialize at the time of declaration
- public readonly int number = 5;
- //You can also initialize through constructor
- public ReadOnlyExample()
- {
- number = 20;
- }
- public ReadOnlyExample(bool IsAnotherInstance)
- {
- number = 100;
- }
- }
- class Program
- {
- static void Main(string[] args)
- {
- ReadOnlyExample readOnlyInstance = new ReadOnlyExample();
- Console.WriteLine(readOnlyInstance.number);
- // You cannot change the value of a readonly variable once it is initialized
- // readOnlyInstance.number = 20;
- ReadOnlyExample readOnlyAnotherInstance = new ReadOnlyExample(true);
- Console.WriteLine(readOnlyAnotherInstance.number);
- Console.WriteLine("Press any key to exist.");
- Console.ReadLine();
- }
- }
- }
OUTPUT:
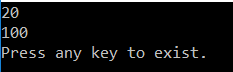
As you can see in the above output, different values coming out of the program’s output for the two different instances of the class. Hence it proves that read-only variables are also immutable values which are known at runtime and do not change their values for the life of the program.
Let us understand Const, Readonly, Static and non-static variable with one example:
- namespace ReadOnlyConstDemo
- {
- class Example
- {
- int x; //Non-static variable
- static int y = 200; //Static Variable
- const float PI = 3.14f; //Const Variable
- readonly bool flag; //Readonly Variable
- public Example(int x, bool flag)
- {
- this.x = x;
- this.flag = flag;
- }
- static void Main(string[] args)
- {
- Console.WriteLine(Example.y);
- Console.WriteLine(Example.PI);
- Example obj1 = new Example(50, true);
- Example obj2 = new Example(100, false);
- Console.WriteLine(obj1.x + " " + obj1.x);
- Console.WriteLine(obj2.flag + " " + obj2.flag);
- Console.WriteLine("Press any key to exist.");
- Console.ReadLine();
- }
- }
- }
OUTPUT:
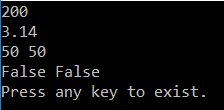
In the next article, I am going to discuss the Properties in C# with some examples. In this article, I try to explain Const and Read-Only in C# step by step with some simple examples. I hope you understood the need and use of Const and Read-Only in C#. Please give your valuable suggestions about this article.
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.