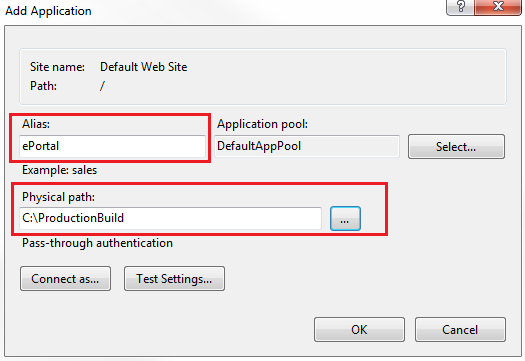
Here are the steps
Step 1 : Build your angular application. If you want to deploy a development build do a development build using the following Angular CLI command. The base-href option on the build command sets the base-href element in index.html to "/ePortal/" instaed of "/". In the IIS server, we will create an application with name "ePortal" in just a bit.ng build --base-href /ePortal/If.