In C#, while loop is used to iterate a part of the program several times. If the number of iteration is not fixed, it is recommended to use while loop than for loop.
Syntax:
Flowchart:
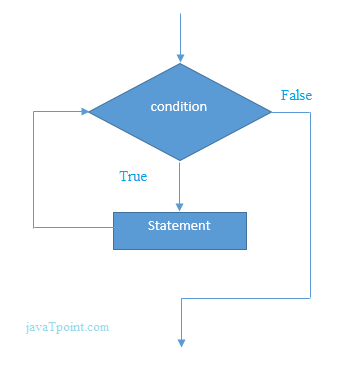
C# While Loop Example
Let's see a simple example of while loop to print table of 1.
Output:
1 2 3 4 5 6 7 8 9 10
C# Nested While Loop Example:
In C#, we can use while loop inside another while loop, it is known as nested while loop. The nested while loop is executed fully when outer loop is executed once.
Let's see a simple example of nested while loop in C# programming language.
Output:
1 1 1 2 1 3 2 1 2 2 2 3 3 1 3 2 3 3
C# Infinitive While Loop Example:
We can also create infinite while loop by passing true as the test condition.
Output:
Infinitive While Loop Infinitive While Loop Infinitive While Loop Infinitive While Loop Infinitive While Loop ctrl+c
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.