The C# do-while loop is used to iterate a part of the program several times. If the number of iteration is not fixed and you must have to execute the loop at least once, it is recommended to use do-while loop.
The C# do-while loop is executed at least once because condition is checked after loop body.
Syntax:
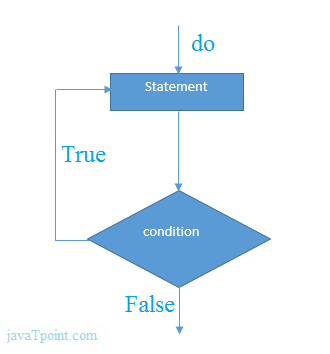
C# do-while Loop Example
Let's see a simple example of C# do-while loop to print the table of 1.
Output:
1 2 3 4 5 6 7 8 9 10
C# Nested do-while Loop
In C#, if you use do-while loop inside another do-while loop, it is known as nested do-while loop. The nested do-while loop is executed fully for each outer do-while loop.
Let's see a simple example of nested do-while loop in C#.
Output:
1 1 1 2 1 3 2 1 2 2 2 3 3 1 3 2 3 3
C# Infinitive do-while Loop
In C#, if you pass true in the do-while loop, it will be infinitive do-while loop.
Syntax:
C# Infinitive do-while Loop Example
Output:
Infinitive do-while Loop Infinitive do-while Loop Infinitive do-while Loop Infinitive do-while Loop Infinitive do-while Loop ctrl+c
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.