In this we will discuss how to control the height and width of Bootstrap form controls.
Bootstrap tutorial for beginners
Bootstrap classes to control the height and width of form controls
Controlling the width of the form controls : In this example we are using predefined bootstrap grid classes to control the width of the form controls.
Controlling the height of the form controls : To control the height of the form controls you may use input-lg or input-sm classes. In the example below, we are not using any of these 2 classes, so we get the default height for the form controls.
To set a larger height for the form controls use input-lg class.
To set a smaller height for the form controls use input-sm class.
To control the height of the form controls and their associated labels on a horizontal form use form-group-lg or form-group-sm classes.
To set a larger height for the form controls and their associated labels use form-group-lgclass
To set a smaller height for the form controls and their associated labels use form-group-sm class
Bootstrap tutorial for beginners
Bootstrap classes to control the height and width of form controls
Class | Purpose |
---|---|
Bootstrap Grid Classes | To control the width of the form controls |
input-lg or input-sm | To control the height of the form controls |
form-group-lg or form-group-sm | To control the height of the form controls and associated labels |
Controlling the width of the form controls : In this example we are using predefined bootstrap grid classes to control the width of the form controls.

<div class="container">
<form>
<div class="row">
<div class="form-group col-xs-3">
<label for="inputName">Name</label>
<input class="form-control" type="text" id="inputName"
placeholder="Full Name" />
</div>
</div>
<div class="row">
<div class="form-group col-xs-3">
<label for="inputDOB">Gender</label>
<select class="form-control">
<option>Male</option>
<option>Female</option>
</select>
</div>
</div>
</form>
</div>
Controlling the height of the form controls : To control the height of the form controls you may use input-lg or input-sm classes. In the example below, we are not using any of these 2 classes, so we get the default height for the form controls.

<div class="container">
<form>
<div class="row">
<div class="form-group col-xs-3">
<label for="inputName">Name</label>
<input class="form-control" type="text" id="inputName"
placeholder="Full Name" />
</div>
</div>
<div class="row">
<div class="form-group col-xs-3">
<label for="inputDOB">Gender</label>
<select class="form-control">
<option>Male</option>
<option>Female</option>
</select>
</div>
</div>
</form>
</div>
To set a larger height for the form controls use input-lg class.

<div class="container">
<form>
<div class="row">
<div class="form-group col-xs-3">
<label for="inputName">Name</label>
<input class="form-control input-lg" type="text" id="inputName"
placeholder="Full Name" />
</div>
</div>
<div class="row">
<div class="form-group col-xs-3">
<label for="inputDOB">Gender</label>
<select class="form-control input-lg">
<option>Male</option>
<option>Female</option>
</select>
</div>
</div>
</form>
</div>
To set a smaller height for the form controls use input-sm class.

<div class="container">
<form>
<div class="row">
<div class="form-group col-xs-3">
<label for="inputName">Name</label>
<input class="form-control input-sm" type="text" id="inputName"
placeholder="Full Name" />
</div>
</div>
<div class="row">
<div class="form-group col-xs-3">
<label for="inputDOB">Gender</label>
<select class="form-control input-sm">
<option>Male</option>
<option>Female</option>
</select>
</div>
</div>
</form>
</div>
To control the height of the form controls and their associated labels on a horizontal form use form-group-lg or form-group-sm classes.
To set a larger height for the form controls and their associated labels use form-group-lgclass
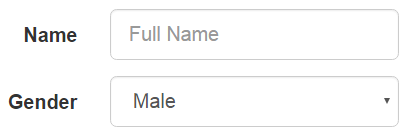
<div class="container">
<form class="form-horizontal">
<div class="form-group form-group-lg">
<label for="inputName" class="control-label col-xs-1">Name</label>
<div class="col-xs-3">
<input class="form-control input-sm" type="text" id="inputName"
placeholder="Full Name" />
</div>
</div>
<div class="form-group form-group-lg">
<label for="inputDOB" class="control-label col-xs-1">Gender</label>
<div class="col-xs-3">
<select class="form-control input-sm">
<option>Male</option>
<option>Female</option>
</select>
</div>
</div>
</form>
</div>
To set a smaller height for the form controls and their associated labels use form-group-sm class

<div class="container">
<form class="form-horizontal">
<div class="form-group form-group-sm">
<label for="inputName" class="control-label col-xs-1">Name</label>
<div class="col-xs-3">
<input class="form-control input-sm" type="text" id="inputName"
placeholder="Full Name" />
</div>
</div>
<div class="form-group form-group-sm">
<label for="inputDOB" class="control-label col-xs-1">Gender</label>
<div class="col-xs-3">
<select class="form-control input-sm">
<option>Male</option>
<option>Female</option>
</select>
</div>
</div>
</form>
</div>