In this article, I am going to discuss HTTP Status Code in ASP.NET Core Web API. Returning the response with a proper status code is the backbone of any restful Web APIs. Now, it is time to learn how can we format the response with the proper response code as per our business requirement.HTTP Status Codes:The HyperText Transport Protocol status code is one of the important components of HTTP Response. The Status code is issued from the server and they give information about the response. Whenever we get any response.
Controller Action Return Types in ASP.NET Core Web API
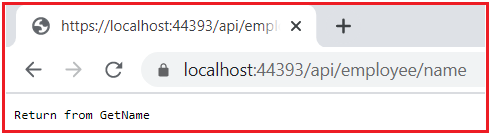
In this article, I am going to discuss the different Controller Action Method Return Types in ASP.NET Core Web API Application with Examples. Please read our previous article, where we discussed Routing in ASP.NET Core Web API. At the end of this article, you will understand what are the different ways to return data from the ASP.NET Core Controller action method.Controller Action Return Types in ASP.NET Core Web APIIn ASP.NET.