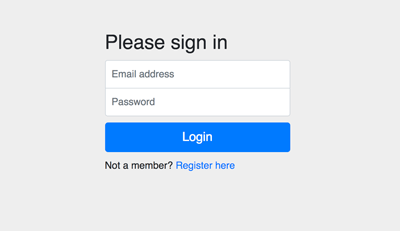
1. Install and Configure Required Modules for React.js
We need additional modules for navigating the views, accessing RESTful API and styling the front end. Type this commands to install the required modules.
npm install --save react-router-dom
npm install --save-dev bootstrap
npm install --save axios
Next, open and edit `src/index.js` then replace all codes with this.
import React from 'react';
import ReactDOM from 'react-dom';
import.