1. Install and Configure Required Modules for React.js
We need additional modules for navigating the views, accessing RESTful API and styling the front end. Type this commands to install the required modules.
npm install --save react-router-dom
npm install --save-dev bootstrap
npm install --save axios
Next, open and edit `src/index.js` then replace all codes with this.
import React from 'react';
import ReactDOM from 'react-dom';
import { BrowserRouter as Router, Route } from 'react-router-dom';
import '../node_modules/bootstrap/dist/css/bootstrap.min.css';
import '../node_modules/bootstrap/dist/css/bootstrap-theme.min.css';
import './index.css';
import App from './App';
import registerServiceWorker from './registerServiceWorker';
import Login from './components/Login';
import Register from './components/Register';
ReactDOM.render(
<Router>
<div>
<Route exact path='/' component={App} />
<Route path='/login' component={Login} />
<Route path='/register' component={Register} />
</div>
</Router>,
document.getElementById('root')
);
registerServiceWorker();
As you see that Login and Register added as the separate component. Bootstrap also included in the import for make the views better. Now, create the new login and register files.
mkdir src/components
touch src/components/Login.js
touch src/components/Register.js
2. Add List of Book to Existing App Component
As you see in the previous step that App component act as home or root page. This component handles the list of books. Open and edit `src/App.js` then replace all codes with this codes.
import React, { Component } from 'react';
import ReactDOM from 'react-dom';
import { Link } from 'react-router-dom';
import axios from 'axios';
class App extends Component {
constructor(props) {
super(props);
this.state = {
books: []
};
}
componentDidMount() {
axios.defaults.headers.common['Authorization'] = localStorage.getItem('jwtToken');
axios.get('/api/book')
.then(res => {
this.setState({ books: res.data });
console.log(this.state.books);
})
.catch((error) => {
if(error.response.status === 401) {
this.props.history.push("/login");
}
});
}
logout = () => {
localStorage.removeItem('jwtToken');
window.location.reload();
}
render() {
return (
<div class="container">
<div class="panel panel-default">
<div class="panel-heading">
<h3 class="panel-title">
BOOK CATALOG
{localStorage.getItem('jwtToken') &&
<button class="btn btn-primary" onClick={this.logout}>Logout</button>
}
</h3>
</div>
<div class="panel-body">
<table class="table table-stripe">
<thead>
<tr>
<th>ISBN</th>
<th>Title</th>
<th>Author</th>
</tr>
</thead>
<tbody>
{this.state.books.map(book =>
<tr>
<td><Link to={`/show/${book._id}`}>{book.isbn}</Link></td>
<td>{book.title}</td>
<td>{book.author}</td>
</tr>
)}
</tbody>
</table>
</div>
</div>
</div>
);
}
}
export default App;
As you can see, there's a call to Book RESTful API when the page is loaded. If API calls response `401` error status then it will be redirected to the login page. We also put a logout button inside the condition where JWT token does not exist in local storage.
3. Add a Component for Login
Previously we have created a Javascript file for login component. Now, open and edit `src/components/Login.js` then add/replace this lines of codes.
import React,{Component} from 'react';
import FaceBookLogin from 'react-facebook-login';
import GoogleLogin from 'react-google-login';
import {PostData} from './postdata';
import {Redirect} from 'react-router-dom';
import Header from '../Results/Header';
import './login.css'
class Welcome extends Component{
constructor(){
super();
this.state={
redirect: false,
datas:[],
mode:'view',username:'',username1:'',email:'',email1:'',password:'',password1:'',telphone:''
};
this.handleSave = this.handleSave.bind(this);
this.handleEdit = this.handleEdit.bind(this);
this.handleEdit3 = this.handleEdit3.bind(this);
this.handleEdit2 = this.handleEdit2.bind(this);
this.handleEdit1 = this.handleEdit1.bind(this);
this.onChange = this.onChange.bind(this);
}
onChange(e){
const state = this.state
state[e.target.name] = e.target.value;
this.setState(state);
}
handleSave() {
this.setState({text: this.state.inputText, mode: 'view'});
}
handleEdit() {
this.setState({mode: 'edit'});
}
handleEdit3() {
const {username,username1,email,email1,password1,telphone,password} = this.state;
this.setState({mode: 'edit1'});
}
handleEdit2(){
var url = document.referrer;
alert(url);
var url1 = url.split('://')[1].split('/')[1]
alert(url1)
const {username,username1,email,email1,password1,telphone,password} = this.state;
fetch('http://localhost:49716/api/UseAuth/login?username=' + username + '&&password='+password)
.then((res) => res.json())
.then((res) => { // responseData = undefined
localStorage.setItem('UserName',res.UserName);
localStorage.setItem('UserID',res.UserLoginId);
alert(res);
this.props.history.push('/'+url1);
});
}
handleEdit1(){
const{username,username1,email,email1,password,password1,telphone} = this.state;
fetch('http://localhost:49716/api/UseAuth/register?customerphoneno=' + telphone + '&&customername='+username1+ '&&password='+password1+ '&&email='+email)
.then((result) =>{localStorage.setItem('jwktoken1',result.data);
this.setState({message:'Registration failed'});this.props.history.push("/login")}).catch((error)=>{if(error.responce.status === 401){this.setState({message: "Registration failed"})}});
}
signup(res,type){
let postData;
if(type === 'facebook' && res.email){
postData ={
name:res.name,
provider:type,
email:res.email,
provider_id:res.id,
token: res.accessToken,
provider_pic: res.picture.data.url
};
}
if(type === 'google' && res.w3.U3){
postData ={
name:res.w3.ig,
provider:type,
email:res.w3.U3,
provider_id:res.El,
token: res.access_token,
provider_pic: res.w3.Paa
};
}
if(postData){
PostData('signup',postData).then((result)=>{
let responseJson = result;
sessionStorage.setItem("userData",JSON.stringify(responseJson));
this.setState({redirect:true});
});
}
else{}
}
renderButton() {
const {username,username1,email,email1,password1,telphone,password} = this.state;
if(this.state.mode === 'view') {
return (
<div>
<div className="row">
<label>Please Login </label></div>
<div className="row">
<label>username</label> <input type="text" value={username} id="username" name="username" placeholder ="username" onChange={this.onChange}/></div>
<div className="row"> <label>password</label><input type="text" id="password" value={password} name="password" placeholder="password" onChange={this.onChange}/></div>
<div className="row">
<button onClick={this.handleEdit2}>
login
</button></div>
<div className="row"> <a onClick={this.handleEdit}>
Register
</a></div>
<div className="row"> <a onClick={this.handleEdit3}>
forgot password
</a> </div>
</div>
);
} else if(this.state.mode === 'edit'){
return (
<div>
<div className="row"> <label>Please Register </label> </div>
<div className="row"> <label>username</label> <input type="text" value={username1} name= "username1" placeholder="Username" onChange={this.onChange}/> </div>
<div className="row"> <label>password</label><input type="text" value={password1} name= "password1" placeholder="Password" onChange={this.onChange}/> </div>
<div className="row"> <label>email ID</label> <input type="email" value={email} name="email" placeholder="Email" onChange={this.onChange}/> </div>
<div className="row"> <label>Phone No</label> <input type="tel" value={telphone} name="telphone" placeholder="telphone" onChange={this.onChange}/> </div>
<div className="row"> <button onClick={this.handleEdit1}>
Register
</button> </div>
<div className="row"> <a onClick={this.handleSave}>
Login
</a> </div>
<div className="row"> <a onClick={this.handleEdit3}>
forgot password
</a> </div>
</div>
);
} else if(this.state.mode === 'edit1'){
return(
<div>
<div className="row"> <label> request for new Password </label> </div>
<div className="row"> <label>email ID</label> <input type="email" value= {email1} id="email1" name="email1" placeholder="Email" onChange={this.onChange} required /> </div>
<div className="row"> <a onClick={this.handleSave}>
Login
</a> </div>
</div>
);
}
}
render(){
if(this.state.redirect || sessionStorage.getItem('userData'))
{
return(<Redirect to={'/home'}/>)
}
const responseFacebook = (response) => {
console.log('facebook console');
console.log(response);
this.signup(response,'facebook');
}
const responseGoogle = (response) =>{
console.log("google console");
console.log(response);
this.signup(response,'google');
}
var shown = {
display: this.state.shown ? "block" : "none"
};
var hidden = {
display: this.state.hidden ? "block" : "none"
}
return(
<div>
<Header/>
<h2 id = "welcome"> </h2>
<div className="container">
<div className="row">
<div className="col-md-3">
<div >
{this.renderButton()}
</div>
</div>
</div>
</div>
</div>
</div>
</div>
);
}
}
export default Welcome;
4. Run and Test the Full Stack Secure Application
It's a time for test the whole full stack secure application. The React.js application will integrate with Express.js by building the React.js application. Type this command to build the React.js application.
npm run-script build
Next, type this command to run the Express.js application.
npm start
Open the browser then point to `localhost:3000`. You will see the default landing page redirected to the login page.
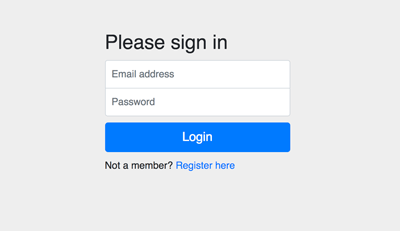
And here's the rest of the register page and main page.
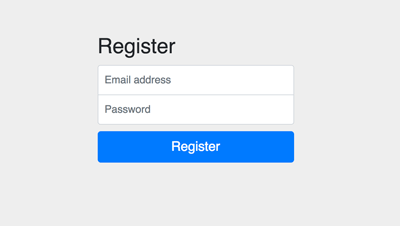
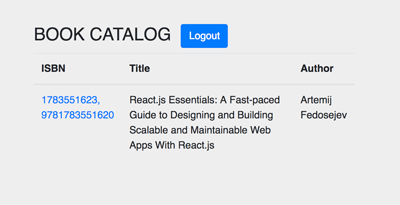
That's it, the complete tutorial of securing full stack web application using Passport.js authentication. If there's something wrong with the steps of the tutorial, you can compare it with the full working source code from our GitHub.
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.