ASP.NET Web API is one of the most powerful recent addition to the ASP.NET framework. Sometimes, you have to post a form data using jQuery-JSON to Web API or MVC method, which have so many input fields. Passing each and every input field data as a separate parameter is not good practice, even when you have a strongly typed-view. The best practice is, pass a complex type object for all the input fields to the server-side to remove complexity.
In this article, I am going to explain to you how can you pass complex types object to the Web API and MVC method to remove complexity at the server-side and make it simple and useful.
Model Classes
Suppose you have the following Product class and repository for a product.
public class Product { public int Id { get; set; } public string Name { get; set; } public string Category { get; set; } public decimal Price { get; set; } } interface IProductRepository { Product Add(Product item); //To Do : Some Stuff } public class ProductRepository : IProductRepository { private List<Product> products = new List<Product>(); private int _nextId = 1; public ProductRepository() { // Add products for the Demonstration Add(new Product { Name = "Computer", Category = "Electronics", Price = 23.54M }); Add(new Product { Name = "Laptop", Category = "Electronics", Price = 33.75M }); Add(new Product { Name = "iPhone4", Category = "Phone", Price = 16.99M }); } public Product Add(Product item) { if (item == null) { throw new ArgumentNullException("item"); } // TO DO : Code to save record into database item.Id = _nextId++; products.Add(item); return item; } //To Do : Some Stuff }
View (Product.cshtml)
<script type="text/javascript"> //Add New Item by Web API $("#Save").click(function () { //Making complex type object var Product = { Id: "0", Name: $("#Name").val(), Price: $("#Price").val(), Category: $("#Category").val() }; if (Product.Name != "" && Product.Price != "" && Product.Category != "") { //Convert javascript object to JSON object var DTO = JSON.stringify(Product); $.ajax({ url: 'api/product', //calling Web API controller product cache: false, type: 'POST', contentType: 'application/json; charset=utf-8', data: DTO, dataType: "json", success: function (data) { alert('added'); } }).fail( function (xhr, textStatus, err) { alert(err); }); } else { alert('Please Enter All the Values !!'); } }); </script> <div> <div> <h2>Add New Product</h2> </div> <div> <label for="name">Name</label> <input type="text" id="Name" title="Name" /> </div> <div> <label for="category">Category</label> <input type="text" id="Category" title="Category" /> </div> <div> <label for="price">Price</label> <input type="text" id="Price" title="Price" /> </div> <br /> <div> <button id="Save">Save</button> <button id="Reset">Reset</button> </div> </div>
Web API Controller
public class ProductController : ApiController { static readonly IProductRepository repository = new ProductRepository(); public Product PostProduct(Product item) { return repository.Add(item); } }
How it works?
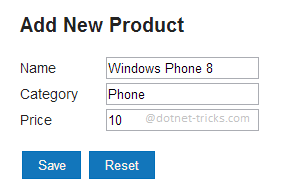
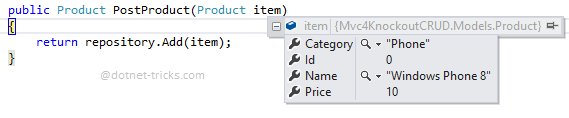
The same thing you have to done with MVC while calling MVC controller method using jQuery-JSON.
What do you think?
I hope you will enjoy the tips while playing with Asp.Net Web API and MVC. I would like to have feedback from my blog readers. Your valuable feedback, question, or comments about this article are always welcome.
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.