In this article, I am going to discuss the Methods and Properties of Console class in C# with some examples. Please read our previous article where we discussed the basic structure of a C# program. As part of this article, I am going to discuss the following pointers related to the Console class in detail.
- What is Console Class in C#?
- Properties of Console Class in C#.
- Methods of Console class in C#.
- Understanding the use of Write and WriteLine method in C#.
- Program to show how to print the value of a variable in a console application.
- Understanding the use of the ReadLine method in C#.
- Program to show the use of BackgroundColor, ForegroundColor and Title properties of Console class.
What is Console Class in C#?
In order to implement the user interface in console applications, Microsoft provided us with a class called Console. The Console class is available in the “System” namespace. This Console class provides some methods and properties using which we can implement the user interface in a console application.
All the properties and methods available in the console class are static. So we can access these members by using the Console class name i.e. we don’t require Console class instance.
Properties of Console Class in C#:
Property | Description |
Title | Specifies the title of the console application |
Background color | Specifies the background color of the text |
Foreground color | Specifies the foreground color of the text |
Cursor size | Specifies the height of the cursor in the console window “1 to 100” |
Methods of Console class in C#:
Method | Description |
Clear() | To clear the screen |
Beep() | Play a beep sound using PC speaker at runtime |
Resetcolor() | Reset the background and foreground color to its default state |
Write(“string”) | Display the specified message on the console window |
WriteLine(“string”) | Same as write method but automatically moves the cursor to the next line after printing the message. |
Write(variable) | Displays the value of the given variable |
WriteLine(variable) | Displays the value of the given variable along with moving the cursor to the next line after printing the value of the variable. |
Read() | Read a single character from the keyboard and returns its ASCII value. The Datatype should be int as it returns the ASCII value. |
ReadLine()
ReadKey()
| Reads a string value from the keyboard and returns the entered value only. As it returns the entered string value so the DataType is going to be a string.
This method reads a single character from the keyboard and returns that character. The Datatype should be int as it returns the ASCII value. It is a STRUCT Data type which is ConsoleKeyInfo.
|
Example: Program to show the use of the Write and WriteLine method:
- namespace FirstProgram
- {
- class Program
- {
- static void Main(string[] args)
- {
- Console.WriteLine("HELLO");
- Console.Write("WELCOME");
- Console.ReadKey();
- }
- }
- }
OUTPUT:

Example: Program to show how to print the value of a variable in a console application.
- namespace FirstProgram
- {
- class Program
- {
- static void Main(string[] args)
- {
- string name = "pranaya";
- Console.WriteLine(name);
- Console.Write("hello " + name);
- Console.ReadKey();
- }
- }
- }
OUTPUT:
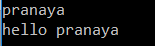
NOTE: Read the values at runtime using the ReadLine() method.
Example: Program to show how to read the value at runtime in a console application.
- namespace FirstProgram
- {
- class Program
- {
- static void Main(string[] args)
- {
- Console.WriteLine("ENTER YOUR NAME");
- String name = Console.ReadLine();
- Console.Write("hello " + name);
- Console.ReadKey();
- }
- }
- }
OUTPUT:
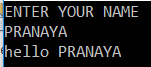
Example: Program to take two numbers as input from the console and then print the summation in the console.
- namespace FirstProgram
- {
- class Program
- {
- static void Main(string[] args)
- {
- int a, b, c;
- Console.WriteLine("ENTER TWO NUMBER");
- a = int.Parse(Console.ReadLine());
- b = Convert.ToInt32(Console.ReadLine());
- c = a + b;
- Console.WriteLine("THE SUM IS :" + c);
- Console.WriteLine("THE SUM IS : " + (a + b));
- Console.ReadKey();
- }
- }
- }
OUTPUT:
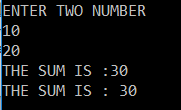
Note: The ReadLine method always accepts the value in the form of a string. So we need to convert the values to the appropriate type. In the above example, we are converting the values to integer type by using int.Parse and Convert.ToInt methods. We will discuss these concepts in details in a later article.
Example: Program to accept employee details like empno, name, salary, address, job and print the accepted information.
- namespace FirstProgram
- {
- class Program
- {
- static void Main(string[] args)
- {
- int EID, SALARY;
- string ENAME, ADDRESS, JOB;
- Console.WriteLine("ENTER THE EMPLOYEE DTAILS");
- Console.WriteLine("ENTER THE EMPLOYEE ID");
- EID = int.Parse(Console.ReadLine());
- Console.WriteLine("ENTER THE EMPLOYEE NAME");
- ENAME = Console.ReadLine();
- Console.WriteLine("ENTER THE EMPLOYEE SALARY");
- SALARY = int.Parse(Console.ReadLine());
- Console.WriteLine("ENTER THE EMPLOYEE ADDRESS ");
- ADDRESS = Console.ReadLine();
- Console.WriteLine("ENTER THE EMPLOYEE JOB");
- JOB = Console.ReadLine();
- Console.WriteLine("\n\n\nTHE EMPLOYEE DETAILS ARE GIVEN BELOW :");
- Console.WriteLine("THE EMPLOYEE ID IS: " + EID);
- Console.WriteLine("THE EMPLOYEE NAME IS: " + ENAME);
- Console.WriteLine("THE EMPLOYEE SALARY IS: " + SALARY);
- Console.WriteLine("THE EMPLOYEE ADDRESS IS: " + ADDRESS);
- Console.WriteLine("THE EMPLOYEE JOB IS: " + JOB);
- Console.ReadKey();
- }
- }
- }
OUTPUT:
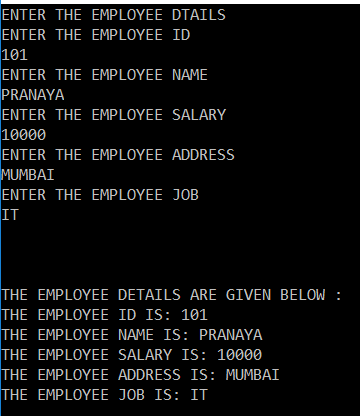
Example:
Program to accept student no, student name, mark1, mark2, mark3 and calculate the total mark and average marks and printing accepted information.
- namespace FirstProgram
- {
- class Program
- {
- static void Main(string[] args)
- {
- int SNO, MARK1, MARK2, MARK3, TOTAL, AVERAGE;
- string SNAME;
- Console.WriteLine("ENTER THE STUDENT DETAILS");
- Console.WriteLine("ENTER THE STUDENT NO");
- SNO = int.Parse(Console.ReadLine());
- Console.WriteLine("ENTER THE STUDENT NAME");
- SNAME = Console.ReadLine();
- Console.WriteLine("ENTER THE MARKS OF 3 SUBJECTS");
- MARK1 = int.Parse(Console.ReadLine());
- MARK2 = int.Parse(Console.ReadLine());
- MARK3 = int.Parse(Console.ReadLine());
- TOTAL = MARK1 + MARK2 + MARK3;
- AVERAGE = TOTAL / 3;
- Console.WriteLine("\n\n\nTHE STUDENT DETAILS ARE GIVEN BELOW :");
- Console.WriteLine("THE STUDENT NO IS: " + SNO);
- Console.WriteLine("THE STUUDENT NAME IS: " + SNAME);
- Console.WriteLine("TOTAL MARKS IS : " + TOTAL);
- Console.WriteLine("AVEARGE MAARK IS: " + AVERAGE);
- Console.ReadKey();
- }
- }
- }
OUTPUT:
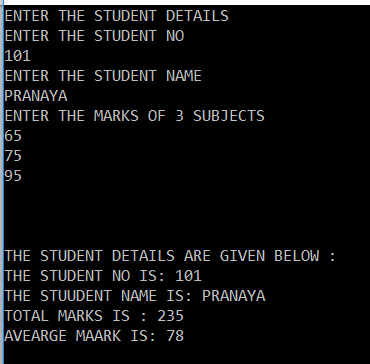
Example:
Program to show the use of BackgroundColor, ForegroundColor and Title properties of Console class in C#.
- namespace FirstProgram
- {
- class Program
- {
- static void Main(string[] args)
- {
- Console.BackgroundColor = ConsoleColor.Blue;
- Console.ForegroundColor = ConsoleColor.White;
- Console.Title = "Understanding Console Class";
- Console.WriteLine("BackgroundColor Blue");
- Console.WriteLine("ForegroundColor White");
- Console.WriteLine("Title Understanding Console Class");
- Console.ReadKey();
- }
- }
- }
OUTPUT:
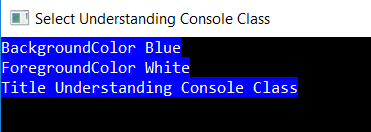
That’s it for today. In the next article, I am going to discuss the Data Types in C# with examples. Here, in this article, I try to explain the methods and properties of Console Class in C# with some simple examples. And I hope you enjoy this article.
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.