In this article, I am going to discuss Inheritance in C# with examples. Inheritance is one of the OOPs principles and as already this principle addresses the extensibility problem. Please read our Class and Object in C# article before proceeding to this article. As part of this article, we are going to discuss the following pointers.
- What is inheritance?
- Types of inheritance in C#
- Why we need inheritance in C#?
- How to make use of inheritance in application development?
What is inheritance?
The process of creating a new class from an existing class such that the new class acquires all the properties and behaviors of the existing class is called inheritance. The properties (or behaviors) are transferred from which class is called the superclass or parent class or base class whereas the class which derives the properties or behaviors from the superclass is known as a subclass or child class or derived class. In simple word, inheritance means to take something that is already made (or available).
Inheritance is the concept which is used for code reusability and changeability purpose. Here changeability means overriding the existed functionality or feature of the object or adding more functionality to the object.
Classification of inheritance supported by C#.NET
C#.NET classified the inheritance into two categories, such as
- Implementation inheritance.
- Interface inheritance
Implementation inheritance: This is the commonly used inheritance. Whenever a class is derived from another class then it is known as implementation inheritance.
Interface inheritance: This type of inheritance is taken from Java. Whenever a class is derived from an interface then it is known as interface inheritance.
Types of inheritance in C#
Inheritance is classified into 5 types. They are as follows.
- Single Inheritance
- Hierarchical Inheritance
- Multilevel Inheritance
- Hybrid Inheritance
- Multiple Inheritance
Single Inheritance: When a class is derived from a single base class then the inheritance is called single inheritance.
Hierarchical Inheritance: Hierarchical inheritance is the inheritance where more than one derived class is created from a single base class.
Multilevel Inheritance: When a derived class is created from another derived class, then that type of inheritance is called multilevel inheritance.
Hybrid Inheritance: Hybrid Inheritance is the inheritance which is the combination of any single, hierarchical and multilevel inheritances.
Multiple Inheritance: When a derived class is created from more than one base class then such type of inheritance is called multiple inheritance. But multiple inheritance is not supported by .net using classes and can be done using interfaces.
Handling the complexity that causes due to the multiple inheritance is very complex. Hence it was not supported in dot net with class and it can be done with interfaces.
Rules to be considered while working with inheritance
Rule1: In inheritance, the constructor of parent class must be accessible to its child class otherwise the inheritance will not possible because when we create the child class object first it goes and calls the parent class constructor so that the parent class variable will be initialized and we can consume them under the child class.
NOTE: The reason why a child class internally calls its parent class constructor is to initialize parent class and can consume them under child class.
Rule2: In inheritance, the child classes can consume the parent class members but parent class does not consume child class members that are purely defined in the child class.
Rule3: Just like the object of a class can be assigned to a variable of same class to make it as a reference, it can also be assigned a variable of its parent to make it as reference so that the reference starts consuming memory of object assigned to it, but now also using that we control access child class pure members.
NOTE: A parent class object can never be assigned to a child class variable. A parent class reference i.e. created by using child class object can be converted back into a child class reference if required by performing an explicit conversion.
Why we need inheritance in C#?
Let us understand why we need inheritance with an example. Assume that a company has n no of branches and asked to computerize branches details of the company, then we create a class like Class Branch having the data member (Data fields or variables) BranchCode, BranchName, and BranchAddress and also methods (functions) like GetBranchData() and DisplayBranchData().
Later after some period of time, the company also asked to computerize employee details of each branch. Then we create a class like Class Employee having the data member EmployeeId, EmployeeName, EmployeeAddress, EmployeeAge and also methods like GetEmployeeData() and DisplayEmployeeData().
If we create two classes without inheritance we need to create the object for every class individually like below
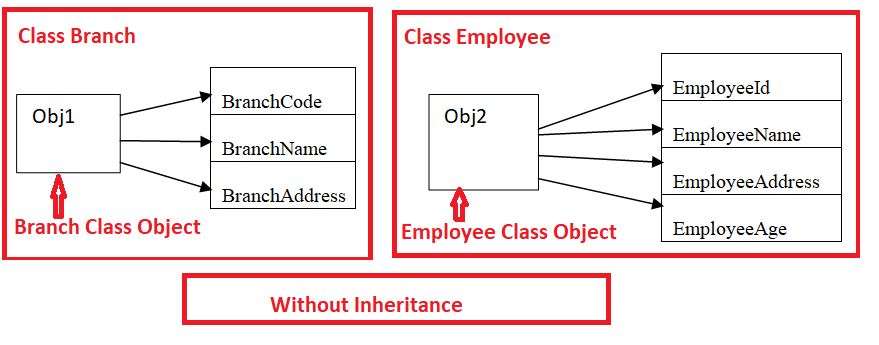
Here Obj1 is the object of Class Branch class and Obj2 is the object of Class Employee. So it becomes very difficult to identify which employee belongs to which branch and integrate the Branch class object with the Employee class object.
So if we derive the Employee class from Branch class we create the object to the derived class Employee then it will represent both classes and will maintain the reference to the members of both base and derived classes like.
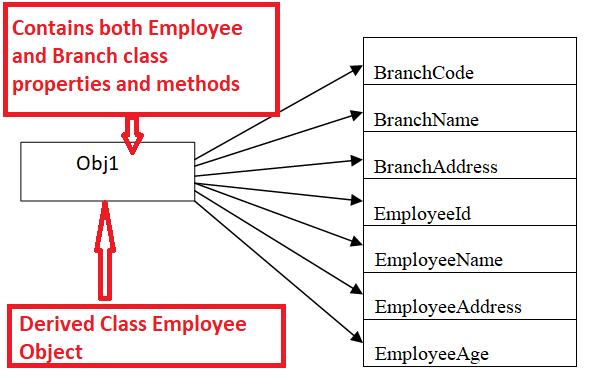
Example:
Let us implement the example we discussed using inheritance. The complete code is given below.
OUTPUT:
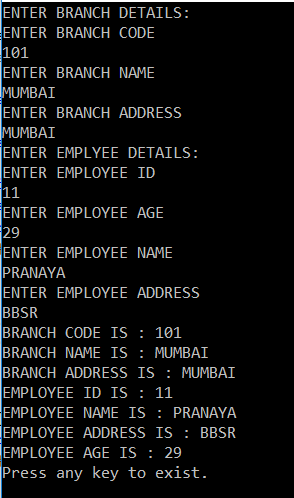
In the above example, we made the functions GetEmployeeData() and DisplayEmployeeData() of class Branch as public because to access from outside the class i.e. from Class Employee.
And the data field BranchCode, BranchName, and BranchAddress are private by default so they are accessible within the same class only.
But if you don’t want to give accessibility of the base class members to the non-derived class (in this case class Program) and would like to give derived class (Employee) then we need to use protected to the members.
And our code would be like as…
How to make use of inheritance in application development?
Generally, when we develop an application we will be following a process as follows.
- Identify the entity that associated in the application
- Identify the attribute that is associated with the application.
- Now separate the attribute of each entity in a hierarchical order without having any duplicate.
- Convert those entities into classes.
Example:
Suppose we are developing an application for school the attributes of the entity will be as following
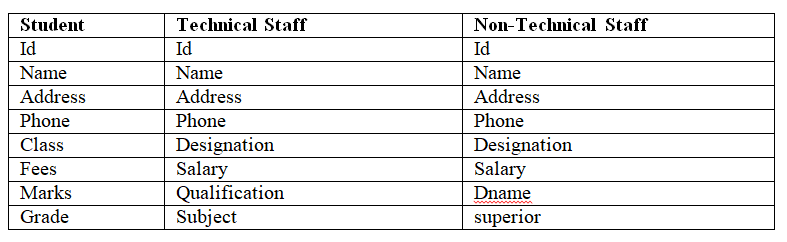
Now separate the attribute of that entity based on the hierarchy as follows.
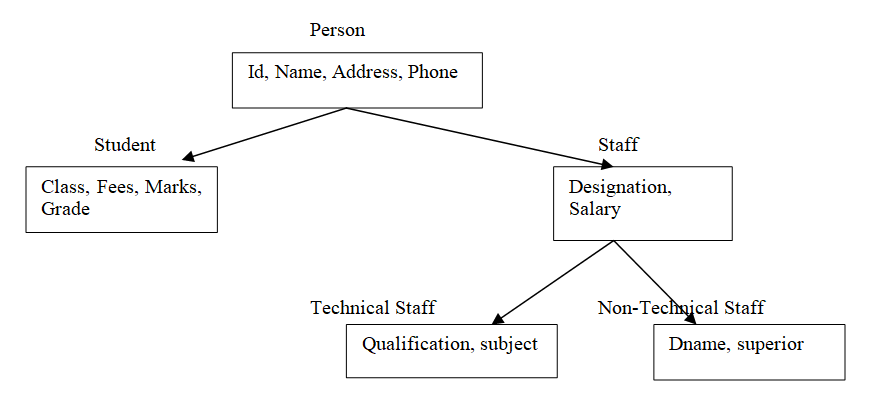
Now define the class representing the entity as following
In the next article, I am going to discuss Interface in C# with some examples. Here, in this article, I try to explain Inheritance in C# step by step with some simple examples. I hope this article will help you with your needs. I would like to have your feedback. Please post your feedback, question, or comments about this article.
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.