In this article, I am going to discuss the Encapsulation in C#. Please read our previous article, before proceeding to this article where we discussed the Access Specifies in C# with examples. Encapsulation is one of the fundamental principles of Object Oriented Programming.
What is Encapsulation in C#?
The process of binding the data and functions together into a single unit (i.e. class) is called encapsulation in C#. Or you can say that the process of defining a class by hiding its internal data member direct access from outside the class and providing its access only through publicly exposed methods (setter and getter methods) or properties with proper validations and authentications is called encapsulation.
The Data encapsulation is also called data hiding because by using this principle we can hide the internal data from outside the class.
How can we implement encapsulation in C#?
In C# encapsulation is implemented
- By declaring the variables as private (to restrict its direct access from outside the class)
- By defining one pair of public setter and getter methods or properties to access private variables.
We declare variables as private to stop accessing them directly from outside the class. The public setter and getter methods or properties are used to access the private variables from outside the class with proper validations. If we provide variables access directly then we cannot validate the data before storing it in the variable.
So the point that you need to remember is by implementing encapsulation in c#, we are protecting or you can say securing the data.
Encapsulation in C# using Accessors and Mutators:
Let us see an example to understand this concept
What are the advantages of providing variable access via setter and getter methods in C#?
We can validate the user given data before it is storing in the variable. In the above program for balance variable –ve value is not allowed. So we can validate the given amount value before storing it in the balance variable. If we provide direct access to balance variable it is not possible to validate the given amount value.
What is the problem if we don’t follow encapsulation in C# while designing a class?
If we don’t use the encapsulation principle while designing the class, then we cannot validate the user given data according to our business requirement as well as it is very difficult to handle future changes.
Let us understand this with an example. Assume in the initial project requirement, the client did not mention that the application should not allow the negative number to store in that variable. So, we give direct access to the variable and the user can store any value to it as shown in the below program.
Output:
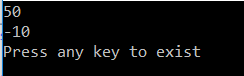
That’s it. It works as expected. Later, in the future, the customer wants that the application should not allow negative numbers. Then we should validate the user given values before storing it into the variable,
Hence the application architecture should be like below
Output:
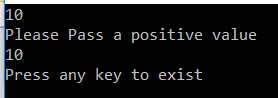
Implementing Encapsulation in C# using Properties:
The Properties are a new language feature introduced in C#. Properties in C# help in protecting a field of a class by reading and writing the values to it. The first method i.e. setter and getter itself is good but Encapsulation in C# can be accomplished much smoother with properties.
Let us understand how to implement Encapsulation in C# using properties with an example
OUTPUT:
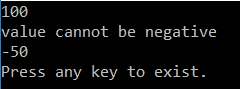
Advantages of Encapsulation:
- Data Hiding: The user will have no idea about the inner implementation of the class. It will not be visible to the user that how the class is stored values in the variables. He only knows that we are passing the values to accessors and variables are getting initialized to that value.
- Increased Flexibility: We can make the variables of the class as read-only or write-only depending on our requirement. If we wish to make the variables as read-only then we have to only use Get Accessor in the code. If we wish to make the variables as write-only then we have to only use Set Accessor.
- Reusability: Encapsulation also improves the re-usability and easy to change with new requirements.
- Testing code is easy: Encapsulated code is easy to test for unit testing.
In the next article, I am going to discuss Abstraction in C# with Examples. Here, in this article, I try to explain the basics of Encapsulation in C# with examples. I hope this article will help you with your need. I would like to have your feedback. Please post your feedback, question, or comments about this article.
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.