The Key attribute can be applied to a property in an entity class to make it a key property and the corresponding column to a PrimaryKey column in the database. The default convention creates a primary key column for a property whose name is Id
or <Entity Class Name>Id
. The Key attribute overrides this default convention.
using System.ComponentModel.DataAnnotations;
public class Student
{
[Key]
public int StudentKey { get; set; }
public string StudentName { get; set; }
}
As you can see in the above example, the
Key
attribute is applied to the StudentKey
property of the Student
entity class. This will override the default conventions and create a primary key column StudentKey
in the Students
table in the database as shown below.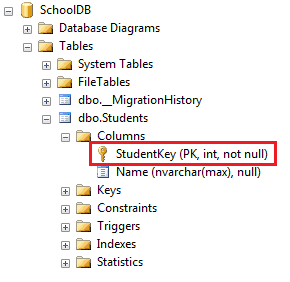
The Key attribute can be applied to a property of any primitive data type except unsigned integers.
EF 6:
In EF 6, the Key attribute along with the
Column
attribute can be applied to multiple properties of an entity class which will create composite primary key columns in the database.
EF Core does not support creating a composite key using the
Key
attribute. You have to use the Fluent API HasKey()
function in EF Core.using System.ComponentModel.DataAnnotations;
public class Student
{
[Key]
[Column(Order=1)]
public int StudentKey { get; set; }
[Key]
[Column(Order=2)]
public int AdmissionNum { get; set; }
public string StudentName { get; set; }
}
The above code creates composite primary key columns
StudentKey
and AdmissionNum
in the Students
table as shown below.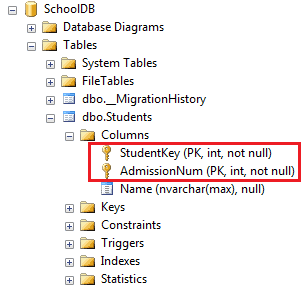
Note: In EF 6, the
Key
attribute creates a PK with an identity column when applied to a single integer type property. The composite key does not create an identity column for the integer property.
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.