Like other programming languages, array in C# is a group of similar types of elements that have contiguous memory location. In C#, array is an object of base type System.Array. In C#, array index starts from 0. We can store only fixed set of elements in C# array.
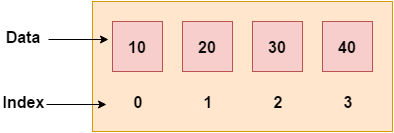
Advantages of C# Array
- Code Optimization (less code)
- Random Access
- Easy to traverse data
- Easy to manipulate data
- Easy to sort data etc.
Disadvantages of C# Array
- Fixed size
C# Array Types
There are 3 types of arrays in C# programming:
- Single Dimensional Array
- Multidimensional Array
- Jagged Array
C# Single Dimensional Array
To create single dimensional array, you need to use square brackets [] after the type.
You cannot place square brackets after the identifier.
Let's see a simple example of C# array, where we are going to declare, initialize and traverse array.
Output:
10 0 20 0 30
C# Array Example: Declaration and Initialization at same time
There are 3 ways to initialize array at the time of declaration.
We can omit the size of array.
We can omit the new operator also.
Let's see the example of array where we are declaring and initializing array at the same time.
Output:
10 20 30 40 50
C# Array Example: Traversal using foreach loop
We can also traverse the array elements using foreach loop. It returns array element one by one.
Output:
10 20 30 40 50
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.