we discussed that, in Angular 2 we have one root injector at the application level plus an injector at every component level as you can see from the diagram below.
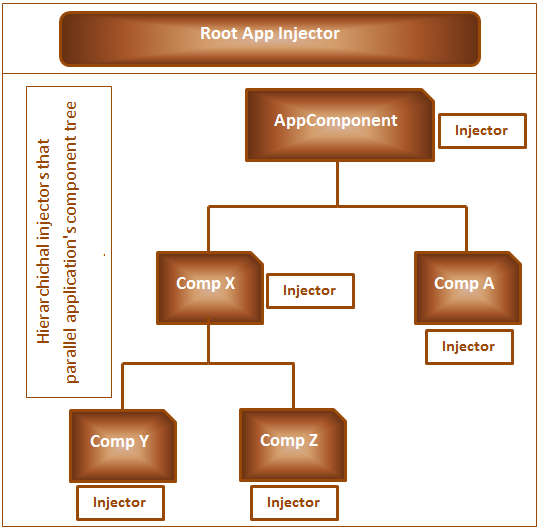
To register a service with the root injector we use providers property of @ngModule decorator and to register a service with the injector at a component level use providers property of @Component decorator.
At the moment in our application we have just one module which is the application root module. In a real world application it is very common to have more than one module. For example, if you are building an HR management system in addition to the root application module (AppModule) we may have feature modules like Employee module, Department Module, Admin Module, Reporting Module etc.
In our previous video we have used the @NgModule() decorator of the root module to register our service with the root injector. We can also use any of the feature module's @NgModule() decorator to register our service with the root injector. Let's prove this.
At the moment in our application we do not have any feature modules. So let's create a simple TestModule and then use that module's @NgModule() decorator to register our service with the root injector.
To create a TestModule
1. Add a new TypeScript file to the "app" folder. Name it "test.module.ts"
2. Copy and paste the following code in it. As you can see from the code below creating a module is very similar to creating a component. To create a component, we first create a class, import @Component() decorator and decorate the class with it.
This makes the TypeScript class an angular component. Along the same lines, to create a module, we first create a class, import @ngModule() decorator and decorate the class with it. This makes the TypeScript class an angular module. So as you can see, Angular provides consistent set of patterns for creating components, pipes, directives and modules.
Notice, we are registering our UserPreferencesService with the root injector using the @NgModule() decorator of this TestModule.
import { NgModule } from '@angular/core';
import { UserPreferencesService } from './employee/userPreferences.service';
@NgModule({
providers: [UserPreferencesService]
})
export class TestModule { }
3. Just like how we have imported angular system modules (like BrowserModule,
FormsModule, HttpModule etc) in our root module(app.module.ts), we need to import the TestModule to be able to use it in our application. So include the required import statement and make the TestModule part of imports array of @NgModule() decorator in the root module (i.e app.module.ts file).
Finally remove "UserPreferencesService" from the providers property. We have already registered our UserPreferencesService with the root injector using the @NgModule() decorator of the TestModule.
import { TestModule } from './test.module';
@NgModule({
imports: [
BrowserModule,
FormsModule,
HttpModule,
TestModule,
RouterModule.forRoot(appRoutes)
],
declarations: [
AppComponent,
EmployeeComponent,
EmployeeListComponent,
EmployeeTitlePipe,
EmployeeCountComponent,
SimpleComponent,
HomeComponent,
PageNotFoundComponent
],
bootstrap: [AppComponent],
providers: [EmployeeService]
})
export class AppModule { }
With all these changes run the application and test. It works exactly the same way as before. So this proves that we can use any module provider property to register a service with the root injector. It can be a feature module or the root module.
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.