Example
For creating a table named "employees".
Create a js file named employees.js having the following data in DBexample folder.
Now open command terminal and run the following command:

Verification
To verify if the table is created or not, use the SHOW TABLES command.
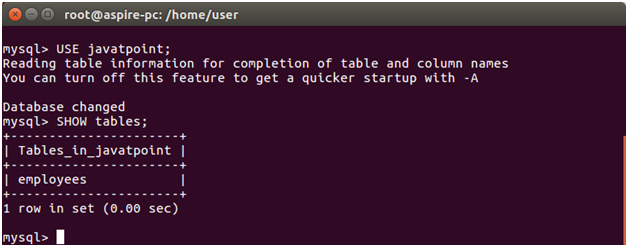
You can also check the structure of the table using DESC command:
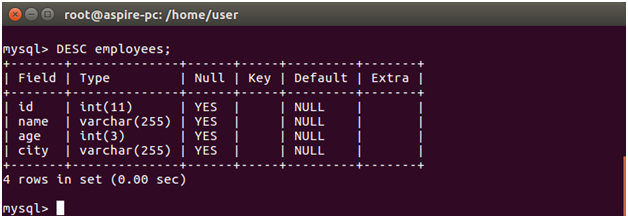
Create Table Having a Primary Key
Create Primary Key in New Table:
Let's create a new table named "employee2" having id as primary key.
Create a js file named employee2.js having the following data in DBexample folder.
Now open command terminal and run the following command:

Verification
To verify if the table is created or not, use the SHOW TABLES command.

You can also check the structure of the table using DESC command to see that id is a primary key :

Add columns in existing Table:
ALTER TABLE statement is used to add a column in an existing table. Take the already created table "employee2" and use a new column salary.
Replace the data of the "employee2" table with the following data:
Now open command terminal and run the following command:

Verification
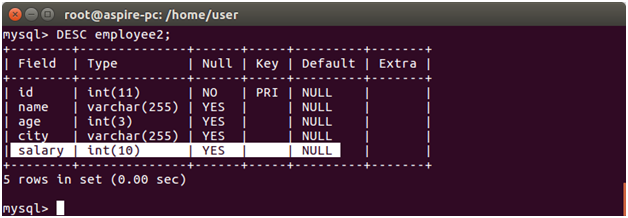
A new column salary is created in the table employee2.
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.