Express.js Middleware are different types of functions that are invoked by the Express.js routing layer before the final request handler. As the name specified, Middleware appears in the middle between an initial request and final intended route. In stack, middleware functions are always invoked in the order in which they are added.
Middleware is commonly used to perform tasks like body parsing for URL-encoded or JSON requests, cookie parsing for basic cookie handling, or even building JavaScript modules on the fly.
What is a Middleware function
Middleware functions are the functions that access to the request and response object (req, res) in request-response cycle.
A middleware function can perform the following tasks:
- It can execute any code.
- It can make changes to the request and the response objects.
- It can end the request-response cycle.
- It can call the next middleware function in the stack.
Express.js Middleware
Following is a list of possibly used middleware in Express.js app:
- Application-level middleware
- Router-level middleware
- Error-handling middleware
- Built-in middleware
- Third-party middleware
Let's take an example to understand what middleware is and how it works.
Let's take the most basic Express.js app:
File: simple_express.js
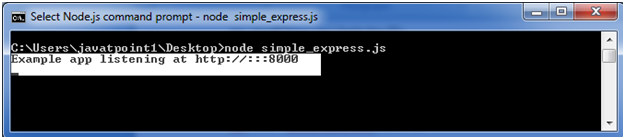
You see that server is listening.
Now, you can see the result generated by server on the local host http://127.0.0.1:8000
Output:
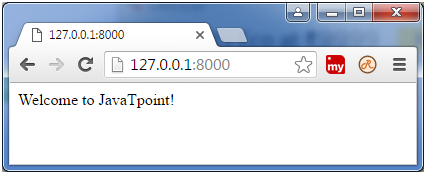
Let's see the next page: http://127.0.0.1:8000/help
Output:
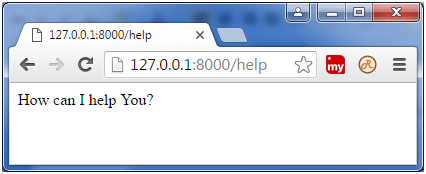

Note: You see that the command prompt is not changed. Means, it is not showing any record of the GET request although a GET request is processed in the http://127.0.0.1:8000/help page.
Use of Express.js Middleware
If you want to record every time you a get a request then you can use a middleware.
See this example:
File: simple_middleware.js

You see that server is listening.
Now, you can see the result generated by server on the local host http://127.0.0.1:8000
Output:
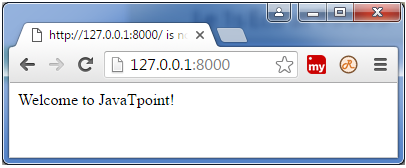
You can see that output is same but command prompt is displaying a GET result.
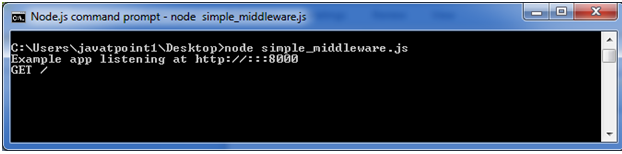
Go to http://127.0.0.1:8000/help


As many times as you reload the page, the command prompt will be updated.
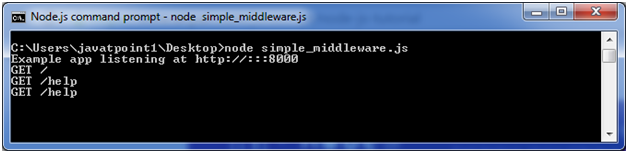
Note: In the above example next() middleware is used.
Middleware example explanation
- In the above middleware example a new function is used to invoke with every request via app.use().
- Middleware is a function, just like route handlers and invoked also in the similar manner.
- You can add more middlewares above or below using the same API.
0 comments:
Post a Comment
Note: only a member of this blog may post a comment.