A “key” is a special string attribute you need to include when
creating lists of elements in React. Keys are used in React to identify
which items in the list are changed, updated or deleted. In other words
we can say that keys are used to give an indentity to the elements in
the lists. The next thing that comes in mind is that what should be good
to be choose as key for the items in lists. It is recommended to use a
string as a key that uniquely identifies the items in the list. Below
example shows a list with string keys:
You can also assign the array indexes as key to the list items. Below example assigns array indexes as key to the elements.
Assigning indexes as keys is highly discouraged
because if the elements of the arrays get’s reordered in future then it
will get confusing for the developer as the key’s for the elements will
also change.
Consider a situation where you have created a separate component for list items and you are extracting list items from that component. In that case you will have to assign keys to the component you are returning from the iterator and not to the list items. That is you should assign keys to <Component /> and not to <li> A good practice to avoid mistake is to keep in mind that anything you are returning from inside of map() function is needed to be assigned key.
Below code shows incorrect usage of keys:
Output:
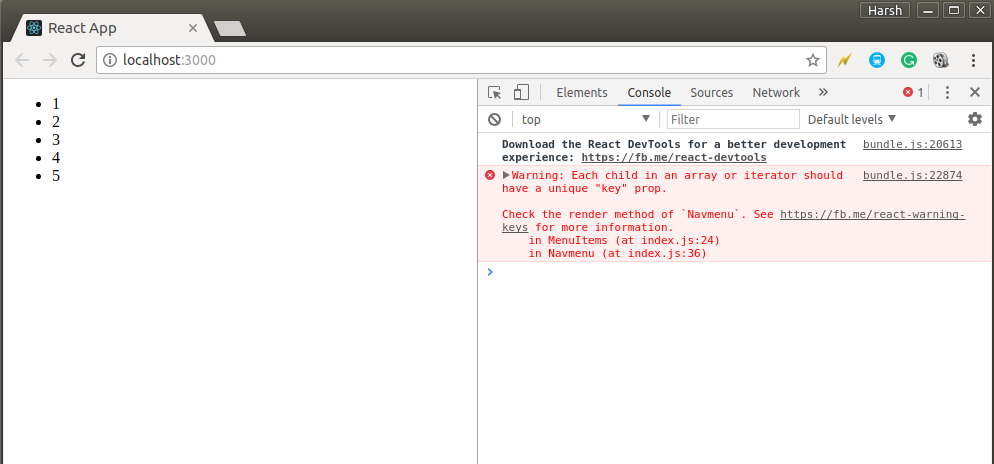
You can see in the above output that the list is rendered successfully but a warning is thrown to the console that the elements inside the iterator are not assigned keys. This is because we had not assigned key to the elements we are returning to the map() iterator.
Below example shows correct usage of keys:
The above code will run successfully without any warning message.
In the below code we have created two different arrays menuItems1 and menuItems2. You can see in the below code that the keys for the first 5 items for both arrays are same still the code runs succesfully without any warning.
Note: Keys are not same as props, only the method of
assigning “key” to a component is same as that of props. Keys are
internal to React and can not be accessed from inside of the component
like props. Therefore, we can use the same value we have assigned to the
Key for any other prop we are passing to the Component.
const numbers = [ 1, 2, 3, 4, 5 ]; const updatedNums = numbers.map((number)=>{ return <li>{ number } </li>; }); |
const numbers = [ 1, 2, 3, 4, 5 ]; const updatedNums = numbers.map((number, index)=>{ return <li>{ number } </li>; }); |
Using Keys with Components
Consider a situation where you have created a separate component for list items and you are extracting list items from that component. In that case you will have to assign keys to the component you are returning from the iterator and not to the list items. That is you should assign keys to <Component /> and not to <li> A good practice to avoid mistake is to keep in mind that anything you are returning from inside of map() function is needed to be assigned key.
Below code shows incorrect usage of keys:
import React from 'react' ; import ReactDOM from 'react-dom' ; // Component to be extracted function MenuItems(props) { const item = props.item; return ( <li> {item} </li> ); } // Component that will return an // unordered list function Navmenu(props) { const list = props.menuitems; const updatedList = list.map((listItems)=>{ return ( ); }); return ( <ul>{updatedList}</ul>); } const menuItems = [1, 2, 3, 4, 5]; ReactDOM.render( , document.getElementById( 'root' ) ); |
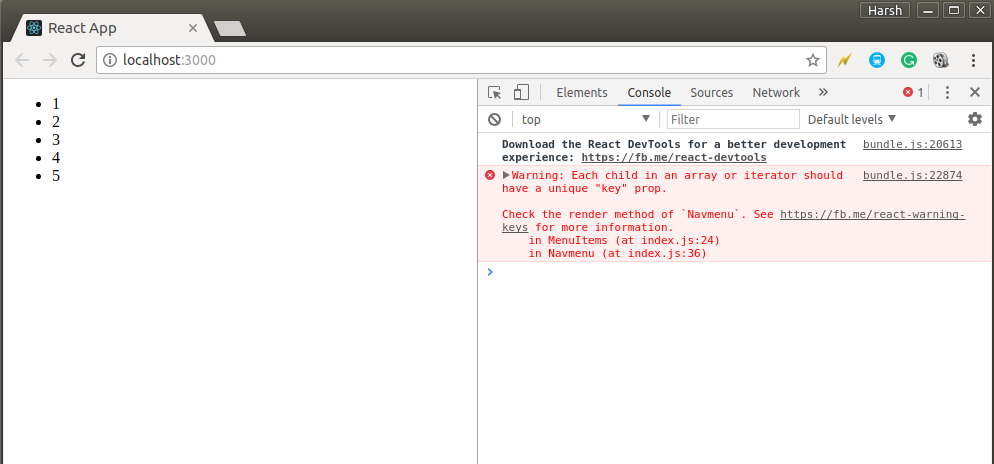
You can see in the above output that the list is rendered successfully but a warning is thrown to the console that the elements inside the iterator are not assigned keys. This is because we had not assigned key to the elements we are returning to the map() iterator.
Below example shows correct usage of keys:
import React from 'react' ; import ReactDOM from 'react-dom' ; // Component to be extracted function MenuItems(props) { const item = props.item; return ( <li> {item} </li> ); } // Component that will return an // unordered list function Navmenu(props) { const list = props.menuitems; const updatedList = list.map((listItems)=>{ return ( ); }); return ( <ul>{updatedList}</ul>); } const menuItems = [1, 2, 3, 4, 5]; ReactDOM.render( , document.getElementById( 'root' ) ); |
Uniqueness of Keys
We have told many times while dicussing about keys that keys assign
to the array elements must be unique. By this we did not meant that the
keys should be globally unique. All the elements in a particular array
should have unique keys. That is, two different arrays can have same set
of keys.In the below code we have created two different arrays menuItems1 and menuItems2. You can see in the below code that the keys for the first 5 items for both arrays are same still the code runs succesfully without any warning.
import React from 'react' ; import ReactDOM from 'react-dom' ; // Component to be extracted function MenuItems(props) { const item = props.item; return ( <li> {item} </li> ); } // Component that will return an // unordered list function Navmenu(props) { const list = props.menuitems; const updatedList = list.map((listItems)=>{ return ( ); }); return ( <ul>{updatedList}</ul>); } const menuItems1 = [1, 2, 3, 4, 5]; const menuItems2 = [1, 2, 3, 4, 5, 6]; ReactDOM.render( <div> </div>, document.getElementById( 'root' ) ); |