1. Full Stack: Install and Create React App
Open the terminal or Node.js command line then go to your MERN projects folder. We will install React app creator for creating a React app easily. For that, type this command.
sudo npm install -g create-react-app
Now, create a React app by type this command.
create-react-app mern-crud
This command will create a new React app with the name `mern-crud` and this process can take sometimes because all dependencies and modules also installing automatically. Next, go to the newly created app folder.
cd ./mern-crud
Now, run the React app for the first time using this command.
npm start
It will automatically open the default browser the point to `http://localhost:3000`, so the landing page should be like this.
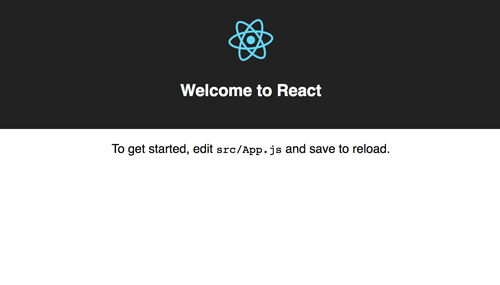
2.Full Stack: Add React Router DOM
Our front end consists of Booklist, detail, create and edit. For navigating between that component, we need to create a route. First, install modules that required by the components.
npm install --save react-route-dom
npm install --save-dev bootstrap
npm install --save axios
Next, open and edit `src/index.js` then replace all codes with this.
import React from 'react';
import ReactDOM from 'react-dom';
import { BrowserRouter as Router, Route } from 'react-router-dom';
import '../node_modules/bootstrap/dist/css/bootstrap.min.css';
import '../node_modules/bootstrap/dist/css/bootstrap-theme.min.css';
import './index.css';
import App from './App';
import registerServiceWorker from './registerServiceWorker';
import Edit from './components/Edit';
import Create from './components/Create';
import Show from './components/Show';
ReactDOM.render(
<Router>
<div>
<Route exact path='/' component={App} />
<Route path='/edit/:id' component={Edit} />
<Route path='/create' component={Create} />
<Route path='/show/:id' component={Show} />
</div>
</Router>,
document.getElementById('root')
);
registerServiceWorker();
You see that Edit, Create and Show added as the separate component. Bootstrap also included in the import to make the views better. Now, create the new edit, create and show files.
mkdir src/components
touch src/components/Create.js
touch src/components/Show.js
touch src/components/Edit.js
3. Full Stack: Add List of Book to Existing App Component
As you see in the previous step that App component act as home or root page. This component handles the list of books. Open and edit `src/App.js` then replace all codes with this codes.
import React, { Component } from 'react';
import ReactDOM from 'react-dom';
import { Link } from 'react-router-dom';
import axios from 'axios';
class App extends Component {
constructor(props) {
super(props);
this.state = {
employees1: []
};
}
componentDidMount() {
axios.get('http://localhost:65388/api/Employees/getemployee')
.then(res => {
this.setState({ employees1: res.data });
console.log(this.state.employees1);
});
}
render() {
return (
<div className="container">
<h4><Link to="/Create"><span className="glyphicon glyphicon-plus-sign" aria-hidden="true"></span> Add Employee</Link></h4>
<table className="table table-stripe">
<thead>
<tr>
<th>EmployeeID</th>
<th>FirstName</th>
<th>LastName</th>
<th>EmpCode</th>
<th>Position</th>
<th>Office</th>
</tr>
</thead>
<tbody>
{this.state.employees1.map(employees =>
<tr>
<td><Link to={`/Show/${employees.EmployeeID}`}>{employees.EmployeeID}</Link></td>
<td>{employees.FirstName}</td>
<td>{employees.LastName}</td>
<td>{employees.EmpCode}</td>
<td>{employees.Position}</td>
<td>{employees.Office}</td>
</tr>
)}
</tbody>
</table>
</div>
);
}
}
export default App;
4.Full Stack: Add Create Components for Add New Book
For add a new book, open and edit `src/components/Create.js` then replace all codes with this codes.
import React, { Component } from 'react';
import ReactDOM from 'react-dom';
import axios from 'axios';
import { Link } from 'react-router-dom';
class Create extends Component {
class Create extends Component {
constructor() {
super();
this.state = {
FirstName: '',
LastName: '',
EmpCode: '',
Position: '',
Office: ''
};
}
onChange = (e) => {
const state = this.state
state[e.target.name] = e.target.value;
this.setState(state);
}
onSubmit = (e) => {
e.preventDefault();
const { FirstName, LastName, EmpCode, Position, Office } = this.state;
axios.post('http://localhost:65388/api/Employees/PostEmployee', { FirstName, LastName, EmpCode, Position, Office })
.then((result) => {
this.props.history.push("/")
});
}
render(){
const { FirstName, LastName, EmpCode, Position, Office } = this.state;
return(
<div>
<h4><Link to="/"><span className="glyphicon glyphicon-th-list" aria-hidden="true"></span>Employees List</Link></h4>
<form onSubmit={this.onSubmit}>
<label>FirstName:
<input type="text" className="form-control" name="FirstName" value={FirstName} onChange={this.onChange} placeholder="FirstName" />
</label>
<label>LastName:
<input type="text" className="form-control" name="LastName" value={LastName} onChange={this.onChange} placeholder="LastName" />
</label>
<label>EmpCode:
<input type="text" className="form-control" name="EmpCode" value={EmpCode} onChange={this.onChange} placeholder="EmpCode" />
</label>
<label>Position:
<input type="text" className="form-control" name="Position" value={Position} onChange={this.onChange} placeholder="Position" />
</label>
<label>Position:
<input type="text" className="form-control" name="Office" value={Office} onChange={this.onChange} placeholder="Office" />
</label>
<button type="submit" className="btn btn-default">Submit</button>
</form>
</div>
)
}
}
export default Create;
5.Full Stack: Add Show Component for Show Book Details
To show book details that listed on the home page, open and edit `src/components/Show.js` then add this lines of codes.
import React, { Component } from 'react';
import axios from 'axios';
import { Link } from 'react-router-dom';
class Show extends Component {
constructor(props) {
super(props);
this.state = {
employees: {}
};
}
componentDidMount() {
axios.get('http://localhost:65388/api/employees1/getemployid?id='+this.props.match.params.id)
.then(res => {
this.setState({ employees: res.data });
console.log(this.state.employees);
});
}
delete(id){
console.log(id);
axios.get('http://localhost:65388/api/employees1/DeleteEmployee?id='+id)
.then((result) => {
this.props.history.push("/")
});
}
render() {
return (
<div className="container">
<div className="panel panel-default">
<div className="panel-heading">
<h3 className="panel-title">
{this.state.employees.EmployeeID}
</h3>
</div>
<div className="panel-body">
<h4><Link to="/"><span className="glyphicon glyphicon-th-list" aria-hidden="true"></span>Employees List</Link></h4>
<dl>
<dt>FirstName:</dt>
<dd>{this.state.employees.FirstName}</dd>
<dt>LastName:</dt>
<dd>{this.state.employees.LastName}</dd>
<dt>EmpCode:</dt>
<dd>{this.state.employees.EmpCode}</dd>
<dt>Position:</dt>
<dd>{this.state.employees.Position}</dd>
<dt>Office:</dt>
<dd>{this.state.employees.Office}</dd>
</dl>
<Link to={`/edit/${this.state.employees.EmployeeID}`} className="btn btn-success">Edit</Link>
<button onClick={this.delete.bind(this, this.state.employees.EmployeeID)} className="btn btn-danger">Delete</button>
</div>
</div>
</div>
);
}
}
export default Show;
In this component, there is two button for edit current book and for delete current book. Delete function included in this component.
6.Full Stack: Add Edit Component for Edit a Book
After the show book details, we need to edit the book. For that open and edit, `src/components/Edit.js` then add this lines of codes.
import React, { Component } from 'react';
import axios from 'axios';
import { Link } from 'react-router-dom';
class Edit extends Component {
constructor(props) {
super(props);
this.state = {
employee: {}
};
}
componentDidMount() {
axios.get('http://localhost:65388/api/Employees/getemployid?id='+this.props.match.params.id)
.then(res => {
this.setState({ employee: res.data });
console.log(this.state.employees);
});
}
onChange = (e) => {
const state = this.state.employee
state[e.target.name] = e.target.value;
this.setState({employee:state});
}
onSubmit = (e) => {
e.preventDefault();
const { FirstName, LastName, EmpCode, Position, Office} = this.state.employee;
axios.put('http://localhost:65388/api/Employees/PutEmployee?id='+this.props.match.params.id, { FirstName, LastName, EmpCode, Position, Office })
.then((result) => {
this.props.history.push("/Show/"+this.props.match.params.id)
});
}
render() {
return (
<div className="container">
<div className="panel panel-default">
<div className="panel-heading">
<h3 className="panel-title">
EDIT Employees
</h3>
</div>
<div className="panel-body">
<h4><Link to={`/show/${this.state.employee.EmployeeID}`}><span className="glyphicon glyphicon-eye-open" aria-hidden="true"></span> Employee List</Link></h4>
<form onSubmit={this.onSubmit}>
<div className="form-group">
<label >FirstName:</label>
<input type="text" className="form-control" name="FirstName" value={this.state.employee.FirstName} onChange={this.onChange} placeholder="FirstName" />
</div>
<div className="form-group">
<label >LastName:</label>
<input type="text" className="form-control" name="LastName" value={this.state.employee.LastName} onChange={this.onChange} placeholder="LastName" />
</div>
<div className="form-group">
<label >EmpCode:</label>
<input type="text" className="form-control" name="EmpCode" value={this.state.employee.EmpCode} onChange={this.onChange} placeholder="EmpCode" />
</div>
<div className="form-group">
<label >Position:</label>
<input type="text" className="form-control" name="Position" value={this.state.employee.Position} onChange={this.onChange} placeholder="Position" />
</div>
<div className="form-group">
<label >Office:</label>
<input type="text" className="form-control" name="Office" value={this.state.employee.Office} onChange={this.onChange} placeholder="Office" />
</div>
<button type="submit" className="btn btn-default">Submit</button>
</form>
</div>
</div>
</div>
);
}
}
export default Edit;
7. Full Stack: Run the React App
Now, we can test our completed app by type this command.
npm run build
npm start
On the browser go to `localhost:3000` then you will see thehome page.
You can navigate and populate the books data.
8. Full Stack: Conclusion
This tutorial aims to make FULL stack as production FULL application, we run Asp.net webapi as the server and React build as the front end. For development purpose, you should separate Asp.net webapi server and React application then make Asp.net webapi CORS enable. Don't worry, you can get the full working source code on our GitHub.