Install the Axios library and send the POST request. If you want to learn about the Axios library, then check out my Getting Started With Axios Promise Based HTTP Client Tutorial Example article.
yarn add axios
# or
npm install axios --save
Now, send the HTTP POST request along with the form data to the node js server.
We will send the data as an object because we have used the body-parser
at the backend to pluck the data from the request and save it in the
database.
Write the following code inside the create.component.js file.
// create.component.js
import React, { Component } from 'react';
import axios from 'axios';
export default class Create extends Component {
constructor(props) {
super(props);
this.onChangePersonName = this.onChangePersonName.bind(this);
this.onChangeBusinessName = this.onChangeBusinessName.bind(this);
this.onChangeGstNumber = this.onChangeGstNumber.bind(this);
this.onSubmit = this.onSubmit.bind(this);
this.state = {
person_name: '',
business_name: '',
business_gst_number:''
}
}
onChangePersonName(e) {
this.setState({
person_name: e.target.value
});
}
onChangeBusinessName(e) {
this.setState({
business_name: e.target.value
})
}
onChangeGstNumber(e) {
this.setState({
business_gst_number: e.target.value
})
}
onSubmit(e) {
e.preventDefault();
const obj = {
person_name: this.state.person_name,
business_name: this.state.business_name,
business_gst_number: this.state.business_gst_number
};
axios.post('http://localhost:4000/business/add', obj)
.then(res => console.log(res.data));
this.setState({
person_name: '',
business_name: '',
business_gst_number: ''
})
}
render() {
return (
<div style={{ marginTop: 10 }}>
<h3>Add New Business</h3>
<form onSubmit={this.onSubmit}>
<div className="form-group">
<label>Person Name: </label>
<input
type="text"
className="form-control"
value={this.state.person_name}
onChange={this.onChangePersonName}
/>
</div>
<div className="form-group">
<label>Business Name: </label>
<input type="text"
className="form-control"
value={this.state.business_name}
onChange={this.onChangeBusinessName}
/>
</div>
<div className="form-group">
<label>GST Number: </label>
<input type="text"
className="form-control"
value={this.state.business_gst_number}
onChange={this.onChangeGstNumber}
/>
</div>
<div className="form-group">
<input type="submit" value="Register Business" className="btn btn-primary"/>
</div>
</form>
</div>
)
}
}
Now, submit the form with proper values and open your browser console panel and see the response.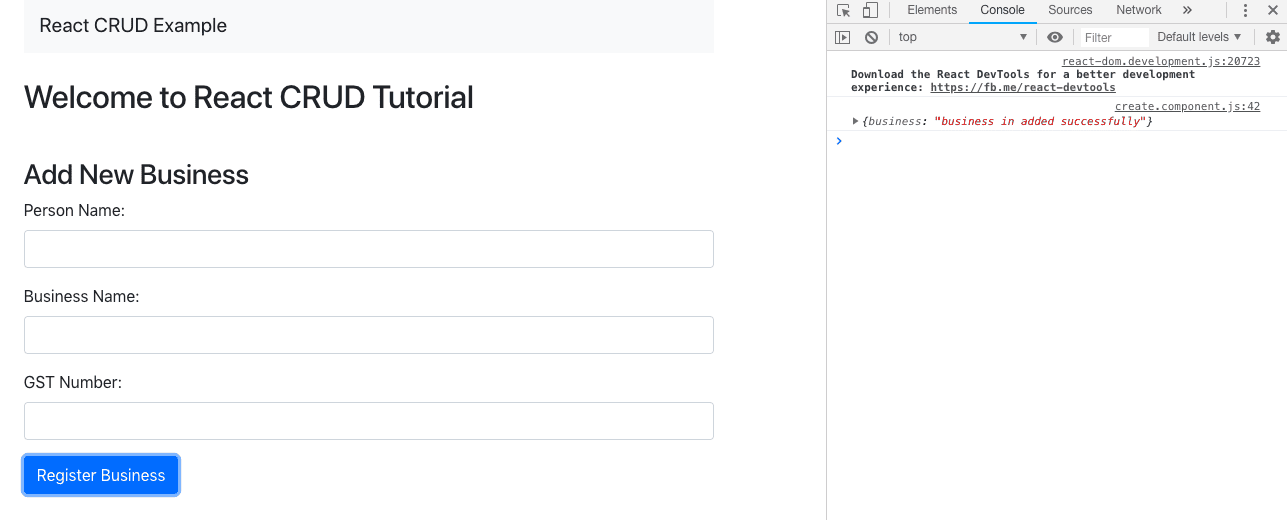
Also, now check inside the mongodb database and see the values.
To see the values inside the database, we can start a mongoshell and look at the values in the database.

So, we can see that our data is added successfully.