What is filter key?
- FilterKeys is a feature of Microsoft Windows. It is an accessibility function that tells the keyboard to ignore brief or repeated keystrokes, in order to make typing easier for users with hand tremors.
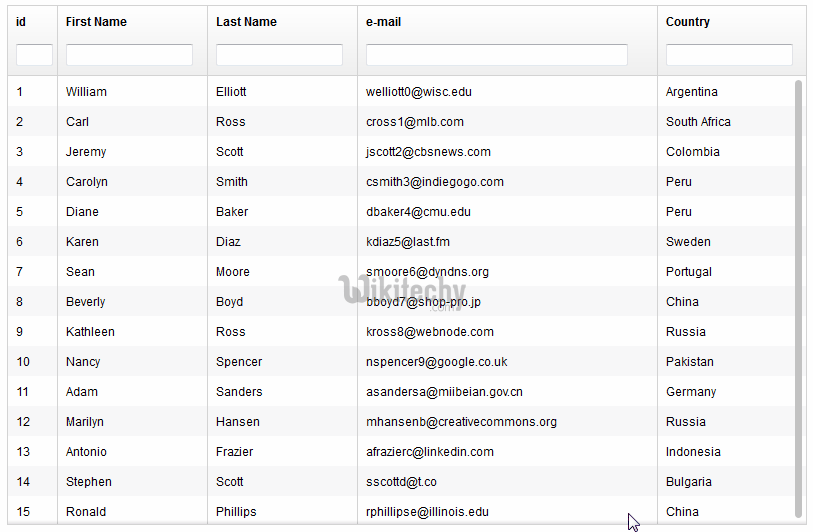
learn react - react filter - react example
Install
$ npm install react-filter
click below button to copy the code. By reactjs tutorial team
Use
var Filter = require('react-filter');
var sample_data = [
{ id : 'id-1', count : 1, date : new Date(2015, 3, 1)},
{ id : 'id-2', count : 2, date : new Date(2015, 3, 2)},
{ id : 'id-3', count : 3, date : new Date(2015, 3, 3)},
{ id : 'id-4', count : 4, date : new Date(2015, 3, 4)}
];
var App = React.createClass({
onChange: fuction(filteredData) {
console.log(filteredData);
},
render: function() {
var opts = {
id : {type: 'text'},
count : {type: 'number'},
date : {type: 'date'}
// key : {opts}
};
return (
<Filter
data={data}
filterOpts={opts}
onChange={this.onChange}
/>
);
}
});
React.render(<App />, document.body);
click below button to copy the code. By reactjs tutorial team
Options
- type {String}-data type
- placeholder {String}-input placeholder
- xs {Number}-value of bootstrap grid system
- xsOffset {Number}-offset value of bootstrap grid system
- perfect- filter perfect matching or not. default false.
{
type : 'text',
placeholder : 'Address',
xs : 3,
xsOffset : 1,
perfect : true
}
click below button to copy the code. By reactjs tutorial team
FilterKeys
- Either an [String] or a String. Will be use by the filter method if no argument is passed there.
Methods
- Return a function which can be used to filter an array. keys can be String, [String] or null.
- If an array keys is an array, the function will return true if at least one of the keys of the item matches the search term.
Article tag : react , react native , react js tutorial , create react app , react tutorial , learn react
Static Methods
- filter(searchTerm, [keys], [{caseSensitive, fuzzy, sortResults}])
- Return a function which can be used to filter an array. searchTerm can be a regex or a String. keys can be String, [String] or null.
- If an array keys is an array, the function will return true if at least one of the keys of the item matches the search term.