Let us understand the difference between Select and SelectMany with an example.
We will be using the following Student class in this demo. Subjects property in this class is a collection of strings.
In this example, the Select() method returns List of List<string>. To print all the subjects we will have to use 2 nested foreach loops.
SelectMany() on the other hand, flattens queries that return lists of lists into a single list. So in this case to print all the subjects we have to use just one foreach loop.
Output:
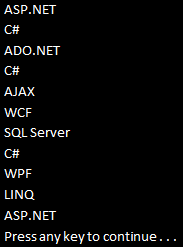
We will be using the following Student class in this demo. Subjects property in this class is a collection of strings.
public class Student
{
public string Name
{ get; set;
}
public string Gender
{ get; set;
}
public List<string> Subjects { get; set; }
public static List<Student> GetAllStudetns()
{
List<Student> listStudents
= new List<Student>
{
new Student
{
Name = "Tom",
Gender = "Male",
Subjects = new List<string> { "ASP.NET",
"C#" }
},
new Student
{
Name = "Mike",
Gender = "Male",
Subjects = new List<string> { "ADO.NET",
"C#", "AJAX" }
},
new Student
{
Name = "Pam",
Gender = "Female",
Subjects = new List<string> { "WCF",
"SQL Server", "C#" }
},
new Student
{
Name = "Mary",
Gender = "Female",
Subjects = new List<string> { "WPF",
"LINQ", "ASP.NET" }
},
};
return listStudents;
}
}
In this example, the Select() method returns List of List<string>. To print all the subjects we will have to use 2 nested foreach loops.
IEnumerable<List<string>> result = Student.GetAllStudetns().Select(s
=> s.Subjects);
foreach (List<string>
stringList in result)
{
foreach (string str
in stringList)
{
Console.WriteLine(str);
}
}
SelectMany() on the other hand, flattens queries that return lists of lists into a single list. So in this case to print all the subjects we have to use just one foreach loop.
IEnumerable<string>
result = Student.GetAllStudetns().SelectMany(s
=> s.Subjects);
foreach (string str in result)
{
Console.WriteLine(str);
}
Output:
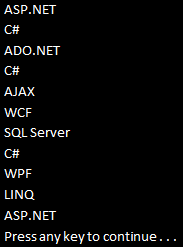